filter() 메서드는 주어진 함수의 테스트를 통과하는 모든 요소를 모아 새로운 배열로 반환합니다. - mdn
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
const result = words.filter(word => word.length > 6);
console.log(result);
// expected output: Array ["exuberant", "destruction", "present"]
다음과 같이 배열에서 원하는 조건의 배열을 리턴하는 filter 함수를 직접 구현해보겠습니다.
const arr = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
먼저 MDN과 같이 임의의 배열을 추가해줍니다.
Array.prototype.reduce2 = function(callback) {
return null;
}
현업에서는 filter2 = () => { ... } 와 같이 함수를 생성하지만 저는 웹브라우저 콘솔에서
테스트를 할것이라 다음과 같이 임의의 함수(filter2)를 생성했습니다.
Array.prototype.filter2 = function(callback) {
console.log(this)
return null;
}
console.log(arr.filter2())
filter2 함수에서 arr에 접근하는 방법은 this를 사용하는 것입니다.
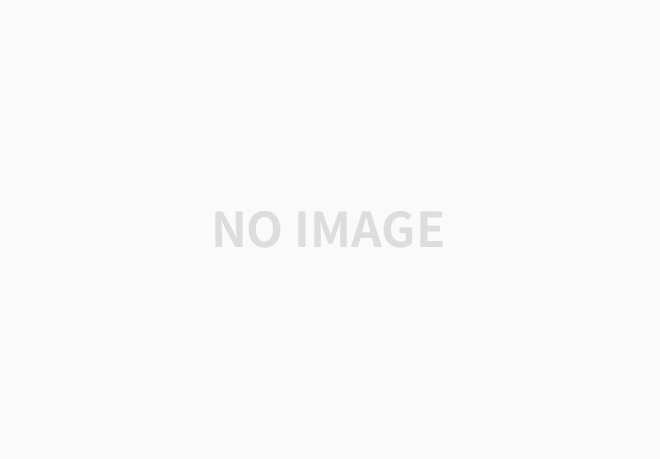
위 코드를 실행하면 다음과 같은 결과를 얻을 수 있습니다.
이제 arr에 접근하는 방법을 알았으니 코드를 작성해보겠습니다.
Array.prototype.filter2 = function(callback) {
const newArray = [];
for(let i = 0; i < this.length; i++){
const result = callback(this[i], i);
if (result){
newArray.push(this[i]);
}
}
return newArray;
}
console.log(
arr.filter2((e,i)=>e.length > 6)
)
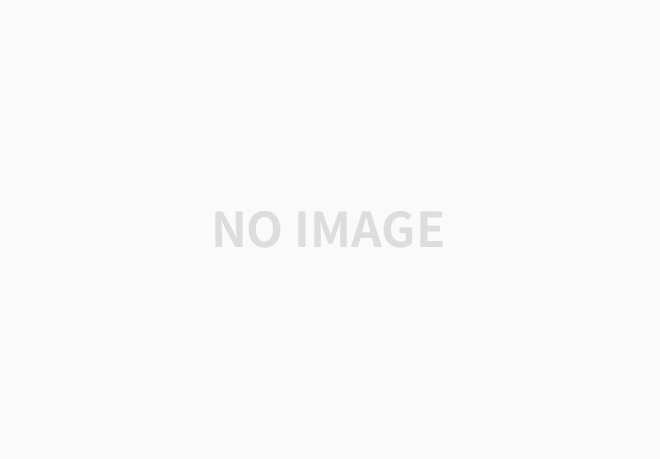
다음과 같이 callback 함수의 e에 arr[index]를 넣고 조건이 true일때만
임의의 배열 newArray에 arr[index]를 푸쉬해서 배열을 만들어서 리턴해줬습니다.
'Study > JS' 카테고리의 다른 글
JS - 배열 순서 바꾸기 (0) | 2023.01.31 |
---|---|
JS - Array.prototype.map() 구현하기 (0) | 2022.11.02 |
JS - Array.prototype.reduce() 구현하기 (0) | 2022.11.02 |
클로저 (Closure) (0) | 2022.10.05 |
진수변환 (0) | 2022.09.29 |